Foss: Java - English
Outline: Getting started with Java Installation * Install jdk from Synaptic Package Manager * Choose openjdk-6-jdk from the list of packages available * Mark it for installation * The installation w..
Basic
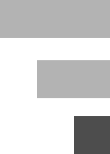
Foss: Java - English
Outline: Java - First Program *write simple java program *print “My First Java Program!” on Console *save the file *file name given to the java file *compile the file *run the file *cor..
Basic
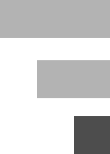
Foss: Java - English
Outline: Installing Eclipse *Install Eclipse on Ubuntu on the Terminal *Set up the proxy on the Terminal *Then fetch the list of all the available softwares *Type sudo apt-get update *Then install..
Basic
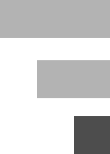
Foss: Java - English
Outline: Getting started with Eclipse *Eclipse is an Integrated Development Environment *It is a tool on which one can write, debug and run java programs easily *Open Dash Home and type Eclipse in the ..
Basic
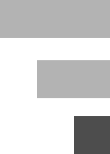
Foss: Java - English
Outline: HelloWorld in Java using Eclipse *Open Eclipse *Create a Java Project named DemoProject *Create a class named DemoClass *Class name and file name will be the same *Eclipse suggests various..
Basic
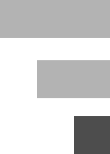
Foss: Java - English
Outline: Errors and Debugging *When writing a Java Program, here is a list of typical errors: *Missing semicolon(;) *Missing double quotes(".") *Mis-match of filename and classname *Typing the prin..
Basic
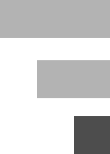
Foss: Java - English
Outline: Programming features of Eclipse *Auto completion *Sets the corresponding closing brace when we open the brace *Provides a drop-down list of methods when you start typing the code *Syntax hig..
Basic
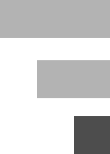
Foss: Java - English
Outline: Numerical datatypes *define datatypes and numerical datatypes *int *float *byte *short *long *double *range of each numerical datatypes *declaration and initialization of numerical..
Basic
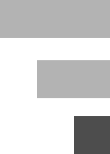
Foss: Java - English
Outline: Arithmetic Operations *Define an operator *Define arithmetic operators *Addition *Subtraction *Multiplication *Division *Modulo *Simple program to demonstrate arithmetic operators ..
Basic
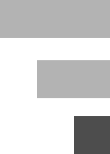
Foss: Java - English
Outline: Strings *char datatype *letter,digit,punctuation marks, tab, or space are all characters *Program explaining the variable and the character data *Introduction to strings *Creating string b..
Basic
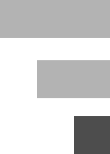
Foss: Java - English
Outline: Primitive Type Conversion *define type conversion or type casting *higher order integer to lower order integer- Explicit type casting *program to show explicit type casting *common mistake i..
Basic
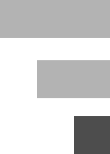
Foss: Java - English
Outline: Relational Operations *boolean datatype *equal to * not equal to *less than *less than or equal to *greater than * greater than or equal to
Basic
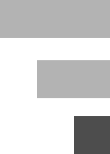
Foss: Java - English
Outline: Logical Operations *use of logical operators *and (&&) operator *example to explain and operator *program to demonstrate and operator *or (||) operator *example to explain or operator ..
Basic
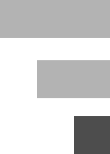
Foss: Java - English
Outline: Outline *Conditional Statements and types of Conditional Statements *Use of if statement *Syntax for if statement *Program using if statement *Use of if else statement *Syntax for if else stat..
Basic
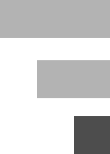
Foss: Java - English
Outline: Outline *explain nested if *nested if syntax *program to demonstrate nested if *explain the control flow of the program *explain ternary operator *syntax for ternary operator *explain the syn..
Basic
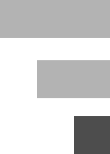
Foss: Java - English
Outline: switch statement define switch case statement compare switch and nested if switch case syntax working of a switch case statement use of keyword switch valid and invalid ..
Basic
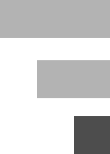
Foss: Java - English
Outline: while loop *Loop control statement *types of loop control statements *Introduction to while loop *syntax of while loop *Program using while loop *Check the output. *Introduction to in..
Basic
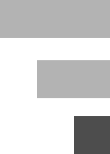
Foss: Java - English
Outline: for loop - introduction to for loop - for loop syntax - loop vaiable - loop condition - loop variable increment or decrement - loop block - flow of loop - advantage of using loop
Basic
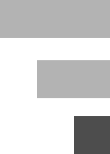
Foss: Java - English
Outline: *define do while *do while syntax *working of do while loop *example of do while loop *explain the do while programming *save, compile and run the program to check the output *how different is..
Basic
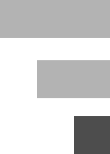
Foss: Java - English
Outline: Introduction to arrays - about arrays. - declare an array - initialize an array - intialization using for loop - index of an array elements - change values of an array - print the value of a..
Basic
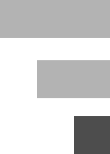
Foss: Java - English
Outline: Array opearations - import java.util.Arrays - use methods from class Arrays - toString() method - sort() method - fill() method - copyOf() method - copyOfRange() method - about parameters f..
Basic
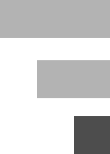
Foss: Java - English
Outline: Creating Class *Whatever we can see in this world are all objects *Objects can be categorized into groups known as class *This is class in real world *Human Being is an example of class in r..
Basic
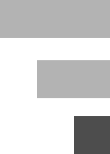
Foss: Java - English
Outline: Creating Object *An object is an instance of a class *Each object consist of state and behavior *Object stores it state in fields or variables *It exposes its behavior through methods *Ref..
Basic
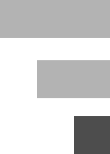
Foss: Java - English
Outline: Instance fields *Also known as non-static fields *Open the TestStudent class which we have created *Access the fields roll_number and name using dot operator *See the output *Initialize th..
Basic
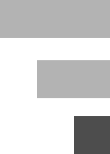
Foss: Java - English
Outline: Methods *method definition * write simple method * method returning value * call a method in another method * flow of the program * call a static method * call a method fro..
Basic
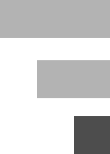
Foss: Java - English
Outline: Default Constructor *what is a constructor? *what is a default constructor? *when is it called? *define a constructor *initialize the variables *call the constructor *difference betwee..
Basic
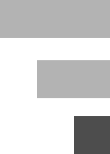
Foss: Java - English
Outline: Parameterized Constructor *What is a parameterized constructor? *create constructor without parameter *create a constructor with parameter *assign values to the variables in the constructor ..
Basic
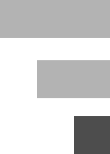
Foss: Java - English
Outline: Using this keyword *this is a reference to the current object *helps to avoid name conflicts *we can use this keyword inside a constructor to call another one *the constructors must be in th..
Basic
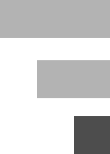
Foss: Java - English
Outline: Non-static block Any code written between two curly brackets Executed for each object that is created Executes before constructor's execution can initialize instance member v..
Basic
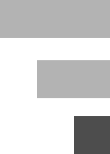
Foss: Java - English
Outline: Constructor overloading *define multiple constructor * what is constructor overloading? *constructor with different number of parameters. *parameters with different datatypes. *how is cons..
Basic
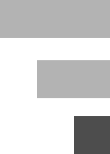
Foss: Java - English
Outline: Method overloading *define multiple methods. *methods with same name. *methods with different number of parameters. *methods with different datatypes of parameter. *what is method overload..
Basic
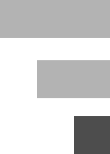
Foss: Java - English
Outline: Taking user input in Java *What is BufferedReader? *Importing three classes from Java.io package *How to take the input from the user? *Syntax to implement BufferedReader *What is InputStr..
Basic
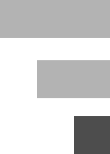
Foss: Java - English
Outline: Definition of subclassing Demo of subclassing using an Employee and Manager class Single inheritance Use of extends keyword Private members in a super class Definition of method overriding Annot..
Intermediate
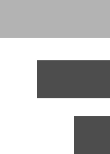
Foss: Java - English
Outline: super keyword Call methods of the super class Constructor of the super class Demo of super keyword using an Employee and Manager class Single inheritance Use of extends keyword Private members ..
Intermediate
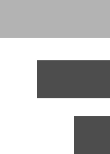
Foss: Java - English
Outline: final keyword What is final keyword and its application? Where final keyword can be declared? final variable final static variables static block final variable as parameter final method priva..
Intermediate
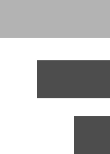
Foss: Java - English
Outline: Polymorphism in Java Run-time polymorphism Virtual Method Invocation Compile-time polymorphism Role of JVM What is IS-A test? What is Static binding? What is Dynamic binding?
Intermediate
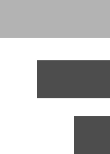
Foss: Java - English
Outline: - Abstract Classes in Java - What are Abstract Methods - What are Concrete Methods - Properties of Abstract Methods and Abstract Classes - How to use Abstract Methods
Intermediate
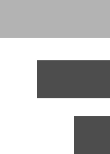
Foss: Java - English
Outline: - Java Interfaces - Implementing Interface - Implementation Classes - Interfaces Vs Abstract classes - Implementing Multiple Interfaces - Usage of Interfaces with an example
Intermediate
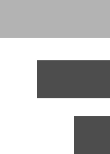